If you’re not familiar with Google Scripts, check it out. Its pretty cool tool to let you automate tasks for various Google services. When I signed up for the LIRR train alert email service, I quickly found out that they come in fairly often. I had a filter that applied a label and skipped inbox which worked well, the only thing is, I would have to go in and manually delete after they accumulated. This is when I used my Google-fu to find a way of automating that task. Once I found Google Scripts, I wrote a short script to delete emails after 24 hours who were tagged with a “LIRR” label. Perfect👌.
So … guess what? You can’t get email notifications for specific folders on Google Drive.
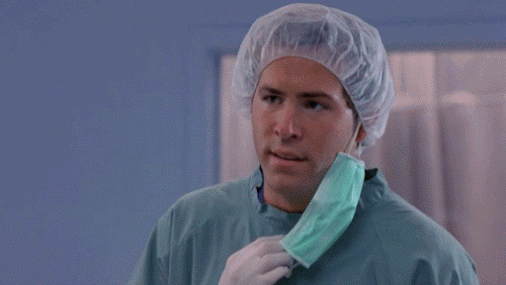
Right Ryan Reynolds, what if we could? What if my buddy Jimmy-Bob wanted to add a few files of shark-kittens to a shared folder of ours and not have to tell me manually(😵). No dice…..UNTIL NOW!
So basic idea: Create a place to a read/write a time stamp. If a file was updated after that timestamp, send an email to a list of email addresses. Simple enough, lets do this 😎.
var folderName = ‘kittens’;
var dataStorefileName = ‘last_updated_kittens_store’;
var emails = [‘firstEmail123@example.com’, ‘otherEmail124@example.com’];
First, we define some hardcoded values where folderName is the folder we are checking, dataStorefileName is the file where we store our last-checked timestamp, and emails is a list emails to send an update to.
var storeFiles = DriveApp.getFilesByName(dataStorefileName);
var currentTime = new Date().getTime();
var lastCheckedDate = new Date(0);
if (storeFiles.hasNext()){
var storeFile = storeFiles.next();
var content = Number(storeFile.getBlob().getDataAsString());
lastCheckedDate = new Date(content);
storeFile.setContent(currentTime);
} else {
DriveApp.createFile(dataStorefileName, currentTime);
return;
}
Next, we check if dataStorefileName exists, if so then we read in the time from the file. When then update the file for the next time the script executes. If the file does not exist, we write a new file with the current time and finish the script (most likely first run).
var emailBody = ‘New files have been added to ‘ + folderName + ‘. Files added: \n\n’;
var folder = DriveApp.getFoldersByName(folderName);
while (folder.hasNext()) {
var kittensFolder = folder.next();
var files = kittensFolder.getFiles();
var updatedFilesFormatted = ‘’;
// If there are files in the folder
if (files.hasNext()){
while (files.hasNext()){
var file = files.next();
// And if the lastUpdated date is greater than the lastCheckedDate
if (file.getLastUpdated() >= lastCheckedDate){
// Format & appened to a String
updatedFilesFormatted = updatedFilesFormatted.concat(file.getName() + ‘\n’);
}
}
// If our formatted string is not empty, email the list to emails
if (updatedFilesFormatted.length > 0){
for (var i = 0; i < emails.length; i++){
GmailApp.sendEmail(emails[i], folderName + ‘ was updated’, emailBody.concat(updatedFilesFormatted));
}
}
}
break;
}
Lastly, we iterate through all the files. If File.getLastUpdated returns a value greater or equal to lastCheckedDate, append the file-name to a string. We then check to see to see if updatedFilesFormatted is empty. If its not, send an email to each of the email address in the emails array.
Thats it! Next step would be the time trigger. You can add one through Resouces > Current Project’s Triggers in the gscript dev window. In this case we would use a time-based trigger with whatever interval you want. This time based trigger means that the script will execute at every interval you specify (i.e. every 1 min, 5 min, 1 hour, etc..).
You can view the full source on my Github. Note, I wrote this in about an hour so its far from perfect, but hey, thats why we iterate.
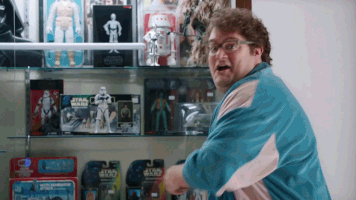